Understanding what Uno Platform is
According to the website (https://platform.uno/), Uno Platform is "the first and only UI Platform for single-codebase applications for Windows, WebAssembly, iOS, macOS, Android and Linux."
That's a complex sentence so let's break down the key elements:
- As a UI platform, it's a way of building applications with a User Interface (UI). This is in contrast to those platforms that are text-based and run from the command line (or equivalent), are embedded in hardware, or are interacted with in other ways, such as by voice.
- Using a single code base means you only need to write code once to have it run on multiple devices and operating systems. Specifically, this means the same code can be compiled for each platform the app will run on. This is in contrast with tools that convert or transpile code into a different programming language before being compiled for another platform. It's also the only code base that's singular, not the output. Some comparable tools create a unique package that runs inside a host application on each OS, or create everything in HTML and JavaScript, and run inside an embedded browser. Uno Platform does neither of these. Instead, it produces native application packages for each platform.
- Windows apps are based on the Universal Windows Platform (UWP) for Windows 10. Work is currently being done at Microsoft to make WinUI 3 the successor to UWP. Uno Platform has partnered with Microsoft to ensure that Uno Platform can easily transition from UWP once WinUI 3 is at a comparable operative level.
- Windows support also includes the Windows Presentation Foundation (WPF), powered by SkiaSharp, for apps that need to run on older versions of Windows (7.1 or 8.1).
- Applications that run in WebAssembly have all their code compiled to run inside a web browser. This means they can be accessed from any device with a compatible browser, without running code on the server.
- By supporting iOS, the apps that are created can run on iPhones and iPads.
- With support for macOS, the apps can run on a MacBook, iMac, or Mac Mini.
- Support for Android applies to phones and tablets running the Android operating system.
- Linux support applies to specific Linux PC equivalent distributions and is powered by SkiaSharp.
Uno Platform does all of the preceding by reusing the tooling, APIs, and XAML that Microsoft created for building UWP apps.
Another way to answer the "what is Uno Platform?" question is that it's a way to write code once and have it run everywhere. The exact definition of "everywhere" is imprecise, as it doesn't include every embedded system or microcontroller capable of running code. Still, many developers and businesses have long had the desire to write code once and run it easily on multiple platforms. Uno Platform makes this possible.
One of the early criticisms of Microsoft's UWP was that it was only universal on Windows. With Uno Platform, developers can now make their UWP apps genuinely universal.
A brief history of Uno Platform
With the varied number of cross-platform tools available today, it's easy to forget how limited the options were back in 2013. At that time, there were no general-purpose tools for easily building native apps that ran on multiple operating systems.
It was at that time that nventive (https://nventive.com/), a Canadian software design and development company, faced a challenge. They had lots of knowledge and experience in building applications for Windows and Microsoft tools, but their customers were also asking them to create applications for Android and iOS devices. Rather than retrain staff or duplicate effort by building multiple versions of the same software for the different platforms, they invented a way to compile the code they wrote for Windows Phone (and later UWP) apps and transfer it to other platforms.
By 2018, it was obvious this approach had been successful for them. They then did the two following things:
- They turned the tool they had created into an open source project, calling it Uno Platform.
- They added support for WebAssembly.
As an open source project, this allowed other developers tackling the same problem to work together. Uno Platform has since seen thousands of contributions from over 200 external contributors, and involvement has been expanded to support more platforms and add additional functionality for the initially supported platforms.
As an open source project, it is free to use. Additionally, it is supported by a company with a business model that was made popular by Red Hat, and has been adopted widely. Usage is free and there is some free public support. However, professional support, training, and custom development are available only through payment.
How Uno Platform works
Uno Platform works in different ways and uses multiple underlying technologies, depending on the platform you're building for. These are summarized in Figure 1.1:
- If you're building an app for Windows 10, Uno Platform does nothing and lets all the UWP tooling compile and execute your app.
- If you're building an app for iOS, macOS, or Android, Uno Platform maps your UI to the native platform equivalents and uses native
Xamarin
libraries to call into the OS it is running on. It produces the appropriate native packages for each OS. - If you're building a WebAssembly app, Uno Platform compiles your code against the
mono.wasm
runtime and maps the UI to HTML and CSS. This is then packaged into a.NET
library that is launched with the Uno Platform web bootstrapper as static web content. - To create Linux apps, Uno Platform converts your code to the
.NET
equivalent and uses Skia to create a version of the UI. It then outputs a.NET5
app that uses GTK3 to present the UI. - Apps for Windows 7 and 8 are created by Uno Platform by wrapping the compiled code in a simple WPF (NETCore 3.1) app that uses SkiaSharp to render the UI.
Refer to the following diagram:
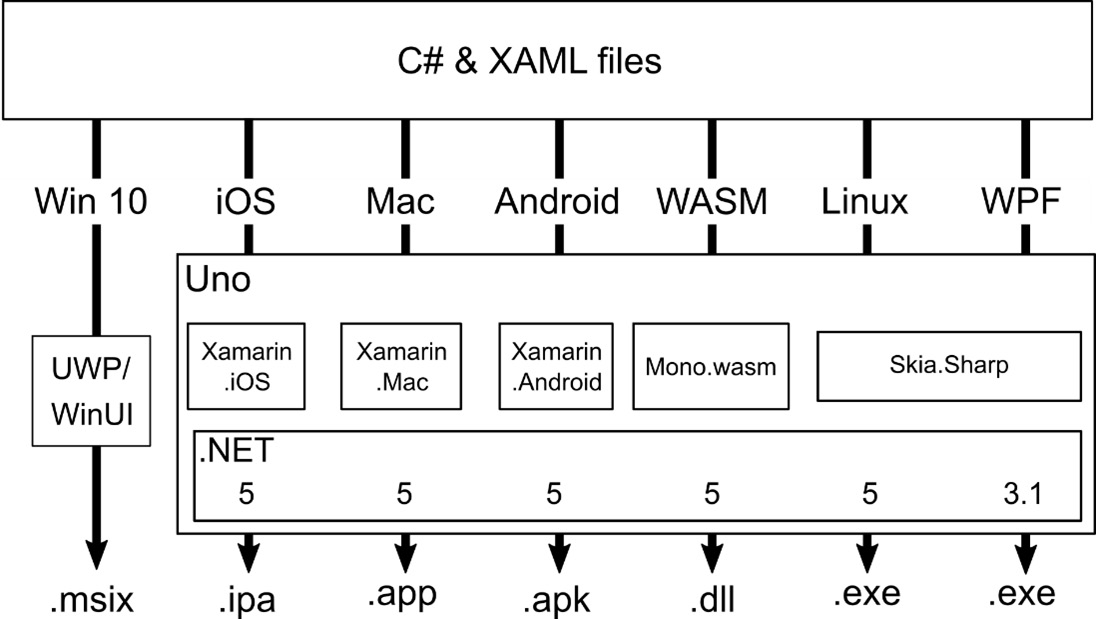
Figure 1.1 – The high-level architecture of Uno Platform
Whichever operating system or platform you're building for, Uno Platform uses the native controls for the platform. This enables your apps to achieve the experience and performance of a fully native app. The exception to this is where it uses SkiaSharp. By using SkiaSharp, Uno Platform draws all UI content on a canvas rather than using platform-native controls. Uno Platform does not add an extra layer of abstraction to the running app (as you might find with cross-platform solutions that use a container, such as an embedded WebView within a shell app).
Uno Platform enables you to do a lot with a single code base. But can it do everything?
Is it a panacea?
The principle of writing code once and running that code everywhere is both powerful and appealing. However, it's necessary to be aware of the following two key points:
- Not all applications should be created for all platforms.
- It's not an excuse for not knowing the platforms the apps will run on.
Additionally, not everything warrants an app. Suppose you just want to share some information that won't be frequently updated. In such a scenario, a website with static web pages would likely be more appropriate.
The lesson just because you can do something doesn't mean you should applies to applications too. When you see how easy it is to create applications that run on multiple platforms, you may be tempted to deploy your applications everywhere you can. Before you do this, there are some important questions you need to ask:
- Is the app wanted or needed on all the platforms? Do people want and need to use it on all the platforms you make it available? If not, you may be wasting effort by putting it there.
- Does the application make sense on all the platforms? Suppose the application has key functionality that involves capturing images while outside. Does it make sense to make it available on a PC or Mac? In contrast, if the application requires the entry of lots of information, is this something people will want to do on the small screen of a mobile phone? Your decision about where to make an application available should be determined by its functionality and the people who will use it. Don't let your decision be based solely on what's possible.
- Can you support it on all platforms? Does the value you gain by making an application available on a platform justify the time and effort in releasing, maintaining, and supporting the application on that platform? If you only have a small number of people use the app on a particular type of device, but they generate many support requests, it's OK to reevaluate your support for such devices.
No technology will render a perfect solution for all scenarios, but hopefully, you can already see the opportunity that Uno Platform provides. Let's now look a bit closer at why and when you might want to use it.