Anatomy of a C++ Application
With a brief understanding of the history of the language, we're going to start our journey by delving into a basic C++ program to see what we're working with. There's no more fitting a start than Hello World!. This famous program prints the words Hello World!
to the console, and has served as the starting point for scores of programmers before you. While basic, it contains all the key components of a C++ application, so will prove a great example for us to de-construct and learn from.
Let's start by taking a look at the program in its entirety:
// Hello world example. #include <iostream> int main() { std::cout << "Hello World!"; return 0; }
Consisting of just seven lines of code, this small program contains everything we need to look at the basic anatomy of a C++ program. We're going to cover each aspect of this program in more detail over the coming chapters, so don't worry if not everything makes perfect sense as we break this program down. The aim here is simply to familiarize ourselves with some core concepts before covering them in more detail as we progress.
Starting from the top, we have a preprocessor directive:
#include <iostream>
Preprocessor directives are statements that allow us to perform certain operations before the program is built. The include
directive is a very common directive that you'll see in most C++ files, and it means "copy here." So, in this case, we're going to copy the contents of the iostream header file into our application, and in doing so, allow ourselves to use input/output functionality it provides.
Next, we have our entry point, main()
:
int main()
The main()
function is where your C++ application will kick-off. All applications will have this function defined and it marks the start of our application—the first code that will be run. This is typically your outer-most loop because as soon as the code in this function is complete, your application will close.
Next, we have an IO statement that will output some text to the console:
std::cout << "Hello World!";
Because we have included the iostream
header at the start of our application, we have access to various input and output functionality. In this case, std::cout
. cout
allows us to send text to the console, so when we run our application, we see that the text "Hello World!"
is printed. We'll cover data types in more detail in the coming chapters.
Finally, we have a return
statement:
return 0;
This signals that we're done in the current function. The value that you return will depend on the function, but in this case, we return 0
to denote that the application ran without error. Since this is the only function in our application, it will end as soon as we return.
And that's our first C++ application; there's not too much to it. From here, the sky is the limit, and we can build applications that are as big and complex as we like, but the fundamentals covered here will stay the same throughout.
Seeing this application typed out is one thing, but let's get it running in our first exercise.
Exercise 1: Compiling Our First Application
In this exercise, we are going to compile and run our first C++ application. We're going to be using an online compiler throughout the course of this book (and the reasons for doing so will be explained after this exercise) but for now, let's get that compiler up and running. Perform the following steps to complete the exercise:
Note
The code file for this exercise can be found here: https://packt.live/2QEHoaI.
- Head to cpp.sh and take a look around. This is the compiler that we'll be using. Once you go to the address, you should observe the following window:
Figure 1.1: C++ shell, the online compiler we'll be using
Options: This allows us to change various compilation settings. We won't be touching this.
Compilation: This shows us the status of our program. If there are any compilation issues, they'll be shown here so we can address them.
Execution: This window is our console, allowing us to interact with the application. We'll input our values here and view the output of the application.
For our first program, we'll run the "
Hello World!
" application we deconstructed in the preceding section. - Type the following code into the code window, replacing all the content that's already there, and then hit
Run
://Hello world example. #include <iostream> int main() { std::cout <<"Hello World!"; return 0; }
As you can see, the console now contains the text Hello World!, meaning our program ran without issue:
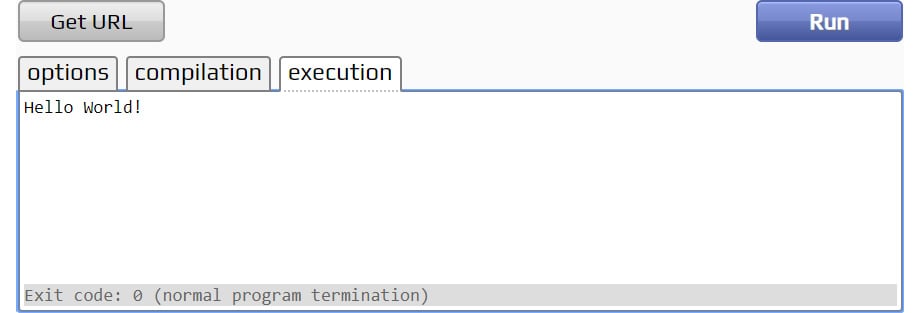
Figure 1.2: Output of our "Hello World" program
Try changing the text to something unique and run the program again.
Note
Here is a partial list of online C++ compilers you can use while working on the exercises. If the one you are using becomes sluggish, or you can't find it at all, try another. Online compilers are useful because they reduce the amount of stuff you have to learn to almost nothing beyond the programming language.
Tutorialspoint C++ compiler: This website allows you to compile a C++ program contained in a single file. It prints error messages from the operating system. You can find it at https://www.tutorialspoint.com/compile_cpp_online.php.
cpp.sh: This website allows you to pick a C++ language version and warning level, and compile a single file. However, it does not print error messages from the operating system. You can find it at http://cpp.sh/.
godbolt compiler explorer: This website allows you to compile a single file on many different compilers and shows the output assembly language; its UI is a little subtle for some tastes. It prints error messages from the operating system. You can find it at https://godbolt.org/.
coliru: This website allows you to compile a single file. It prints error messages from the operating system. You can find it at http://coliru.stacked-crooked.com/.
repl.it: This website allows you to compile multiple files. You can find it at https://repl.it/languages/cpp.
Rextester: This website lets you compile a single file using Microsoft Visual C++. You can find it at https://rextester.com/.
C++ Build Pipeline
Before we go any further, let's take a moment to discuss the build pipeline. This is the process that turns the code that we write into an executable that our machines are capable of running. When we write our C++ code, we're writing a highly abstracted set of instructions. Our machines don't natively read C++ as we do, and likewise, they're unable to run our C++ files as we write them. They first have to be compiled into an executable. This process consists of a number of discrete steps and transforms our code into a more machine-friendly format along the way:
- Preprocessor: As the name implies, it runs through our code before it's compiled, resolving any preprocessor directives that we may have used. These include things such as
include
statements, which we saw previously, and others such as macros and defines that we'll look at later in this chapter.Our files are still human-readable at this point. Think of the preprocessor as a useful editor that will run through your code, doing all the little jobs you've marked, preparing our code for the next step—the compiler.
- Compilation: The compiler takes our human-readable files and converts them into a format that the computer can work with—that is, binary. These are stored in object files that end with
.o
or.obj
, depending on the platform. Consider the smallHello World !
application we dissected earlier. All that code lives in a single file, main.cpp. If we were to pass that to a compiler, we would get back main.o; an object file containing the binary version of our source code that the machine can run. This isn't quite ready to run yet, and you can't directly execute an object file. Before we can execute our application, we need to look at the final step of the pipeline—the linker. - Linker: The linker is the last step in producing our executable. Once the compiler has turned our source code into binary objects, the linker comes through and links them all together, putting together our final executable.
The aforementioned steps have been visualized in the following process flow diagram:
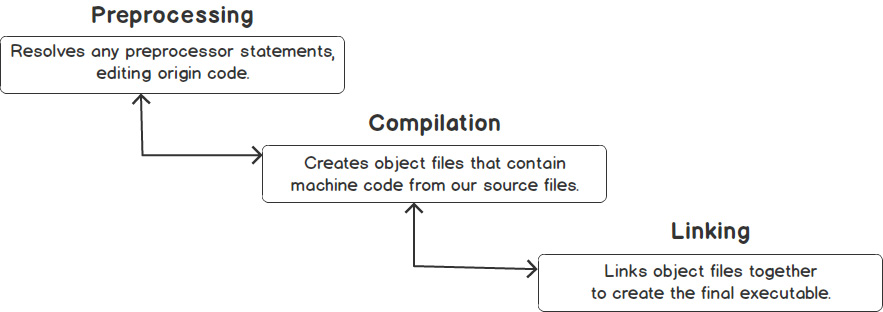
Figure 1.3: The various step of compilation and linking
These three steps are what every C++ application goes through, be it a single-file program such as the "Hello World!" program we've already discussed, or a multi-thousand-file application that you might see in real-world applications; these fundamental steps remain the same.
Different operating systems have different toolsets that perform these actions, and covering them all would not only take focus away from writing C++ itself, but potentially create different experiences, depending on the setup, especially because they're always changing. That's why in this book we'll be using an online compiler. Not only can we jump straight into writing code, but we can be sure that everyone will have the same results.
This overview of these processes has hopefully provided a solid overview of the fundamentals, so that when you do look to compile your applications in the future, the process will be familiar and you'll understand what's going on behind the scenes.