About the Book
C++ is the backbone of many games, GUI-based applications, and operating systems. Learning C++ effectively is more than a matter of simply reading through theory, as the real challenge is understanding the fundamentals in depth and being able to use them in the real world. If you're looking to learn C++ programming efficiently, this Workshop is a comprehensive guide that covers all the core features of C++ and how to apply them. It will help you take the next big step toward writing efficient, reliable C++ programs.
The C++ Workshop begins by explaining the basic structure of a C++ application, showing you how to write and run your first program to understand data types, operators, variables and the flow of control structures. You'll also see how to make smarter decisions when it comes to using storage space by declaring dynamic variables during program runtime.
Moving ahead, you'll use object-oriented programming (OOP) techniques such as inheritance, polymorphism, and class hierarchies to make your code structure organized and efficient. Finally, you'll use the C++ standard library's built-in functions and templates to speed up different programming tasks.
By the end of this C++ book, you will have the knowledge and skills to confidently tackle your own ambitious projects and advance your career as a C++ developer.
About the Chapters
Chapter 1, Your first C++ Application, will equip you with the fundamental tools and techniques required to get started building basic C++ applications.
Chapter 2, Control Flow, presents various tools and techniques that are used to control the flow of execution throughout applications.
Chapter 3, Built-in Data Types, presents the built-in data types provided by C++, including their fundamental properties and use within vectors and arrays. These are then utilized in the creation of a real-world sign-up application.
Chapter 4, Operators, presents a variety of operators provided by C++, describing what they do and how they can allow us to manipulate our data.
Chapter 5, Pointers and References, presents a variety of operators provided by C++, describing what they do and how they can allow us to manipulate our data.
Chapter 6, Dynamic Variables, introduces dynamic variables – that is, variables that can be created when needed and can hold an arbitrarily large amount of data that is limited only by the memory that is available.
Chapter 7, Ownership and Lifetime Of Dynamic Variables, makes the use of pointers in C++ programs safer and easier to understand.
Chapter 8, Classes and Structs, presents the fundamentals of structs and classes with the aid of practical examples and exercises.
Chapter 9, Object-Oriented Principles, presents best practices for designing classes and will give you an overview of abstraction and encapsulation, where to use them, and how they can benefit your custom C++ types.
Chapter 10, Advanced Object-Oriented Principles, presents a number of advanced object-oriented principles, including inheritance and polymorphism, that will allow us to build more dynamic and powerful C++ applications.
Chapter 11, Templates, covers an overview of templates and gives some examples of how they can be used and where and teaches you how to implement template types and functions.
Chapter 12, Containers and Iterators, provides an overview of using the containers and iterators provided by the C++ standard library.
Chapter 13, Exception Handling, covers exception handling, the mechanism used by C++ for reporting and recovering from unexpected events in a program.
Conventions
Code words in text are shown as follows: "The #include <typeinfo>
line gives access to the name of the passed-in type through the name()
function."
A block of code is set as follows:
#include <iostream> #include <string.h> using namespace std; template<typename T> bool compare(T t1, T t2) { return t1 == t2; }
New terms and important words are shown like this: "In the previous chapters, object-oriented programming (OOP) was introduced, along with examples and use cases."
Long code snippets are truncated and the corresponding names of the code files on GitHub are placed at the top of the truncated code. The permalinks to the entire code are placed below the code snippet. It should look as follows:
Example09_1.cpp
23 string getName() 24 { 25 return m_trackName; 26 } 27 28 void setName(string newTrackName) 29 { 30 // if S-Club is not found set the track name - otherwise do nothing 31 if (newTrackName.find("S-Club") == string::npos) 32 { 33 m_trackName = newTrackName; 34 } 35 } 36 37 void setLength(float newTrackLength) 38 { 39 if (newTrackLength < MAX_TRACK_LENGTH && newTrackLength > 0) 40 // no prog metal for us! 41 { 42 m_lengthInSeconds = newTrackLength; 43 } 44 }
The complete code for this example can be found at: https://packt.live/2DLDVQf
Before You Begin
There are many tools we can use to compile our C++ programs, too many to cover here, so here are some recommendations and a guide on getting started:
Online Compilers
cpp.sh is an online C++ compiler and the one the authors extensively used in this book. Visit cpp.sh and ensure the options are set up as shown:
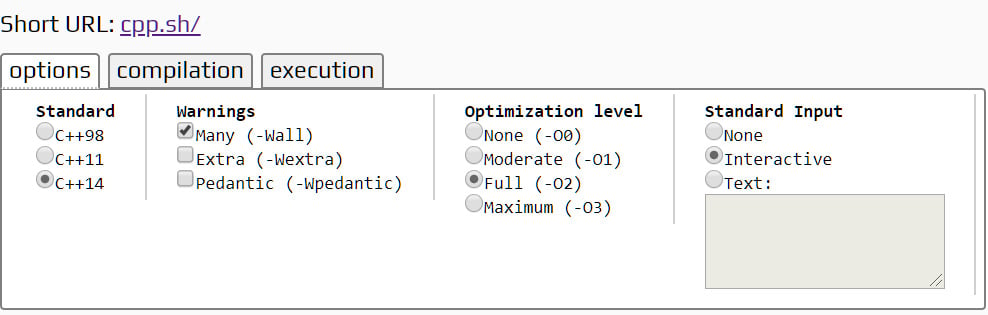
Figure 0.1: Screenshot of the cpp.sh online compiler
That is all we need to do to get started using this compiler. Simply write out your code and hit the run button. Any errors will appear in the compilation tab, and interactive standard input and output will be located on the execution tab. Here is a partial list of online C++ compilers you can use while working on the exercises. If the one you are using becomes sluggish, or you can't find it at all, try another:
Tutorialspoint C++ compiler: This website allows you to compile a C++ program contained in a single file. It prints error messages from the operating system. You can find it at https://www.tutorialspoint.com/compile_cpp_online.php.
godbolt compiler explorer: This website allows you to compile a single file on many different compilers and shows the output assembly language; its UI is a little subtle for some tastes. It prints error messages from the operating system. You can find it at https://godbolt.org/.
coliru: This website allows you to compile a single file. It prints error messages from the operating system. You can find it at http://coliru.stacked-crooked.com/.
repl.it: This website allows you to compile multiple files. You can find it at https://repl.it/languages/cpp.
Rextester: This website lets you compile a single file using Microsoft Visual C++. You can find it at https://rextester.com/.
Installing the Code Bundle
Download the code files from GitHub at https://github.com/PacktWorkshops/The-CPP-Workshop and place them in a new folder called C:\Code
. Refer to these code files for the complete code bundle.
If you face any trouble with installation or with getting the code up and running, please reach out to the team at [email protected].