A picture is worth a thousand words, and this is, after all, a book on 3D graphics. So, let's get started with the fun stuff! Two technologies will be demonstrated: WebGL and X3D. WebGL is related to X3D, but X3D is better to demonstrate simple objects. Since we will be building the Solar System, X3D is better in order to show the three rotations of the Earth—the 24-hour day rotation, the seasonal tilts, and the 365.25 annual rotation around the Sun. The Moon is a child of the Earth. Wherever the Earth goes, the Moon follows with its own independent rotation around the Earth. To assist the parsing of these X3D files, we shall use X3DOM (X3D with the Document Object Model (DOM))—a publicly available program. WebGL, by itself, is ideal to display the 3D mesh, whereas X3D better represents the connection between objects. For example, an X3D file can show the hierarchy of an animated hand rotating at the wrist, which is connected to the lower arm that rotates at the elbow, and then the shoulder.
Programming tradition states that the first program shall be called "Hello World" and simply displays these words. 3D also has "Hello World"; however, it displays the simplest 3D object—a box.
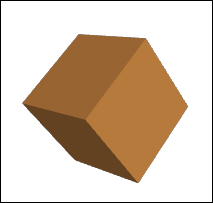
A <Box>
node is one of the several simple primitives that are included in X3D; the other shape nodes are <Cone>
, <Cylinder>
, and <Sphere>
. While WebGL is JavaScript programming, X3D looks like HTML tags. So, angular brackets, <
and >
, will often be used to describe X3D.
However, we will not cover basic HTML tags, CSS, or JavaScript. There is too much to cover in 3D, and these are better addressed online. A good source for basic HTML and web programming can be found at http://www.w3schools.com/. Here is our first <Box>
shape node used in the following code:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="X-UA-Compatible" content="chrome=1" /> <meta http-equiv="Content-Type" content="text/html;charset=utf-8" /> <title>WebGL Hotshots - chapter 01</title> <link rel="stylesheet" type="text/css" href="x3dom.css"/> <script type="text/javascript" src="x3dom.js"></script> </head> <body> <X3D xmlns=http://www.web3d.org/specifications/x3d-namespace width="400px" height="400px"> <Scene> <Shape> <Appearance> <Material diffuseColor='0.9 0.6 0.3'/> </Appearance> <Box/> </Shape> </Scene> </X3D> </body> </html>
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
Between the <head>
and </head>
tags are two references that link this xhtml
file to the X3DOM JavaScript code, x3dom.js
. This code parses the X3D file and loads the data onto the graphics card using the OpenGL commands. So, it takes care of a lot of low-level coding, which we will look at later using WebGL. Also, the x3dom.css
file sets some parameters similar to any CSS file. Thus, a basic knowledge of HTML is helpful to develop WebGL. Some of the other tags in the preceding code relate to the validation process of all XML documents such as the DOCTYPE
information in the preceding code.
The heart of the matter begins with the <X3D>
tag being embedded into a standard XHTML
document. It can also include X3D version information, width and height data, and other identifications that will be used later. There are also a set of <Scene>
and </Scene>
tags within which all the 3D data will be contained.
The <Shape>
tags contain a single 3D mesh and specify the geometry and appearance of the 3D mesh. Here, we have a single <Box/>
tag and the <Appearance>
tag, which specifies either a texture map and/or a <Material>
tag that includes several properties, namely, the diffuse color that will be blended with the color of our scene's lights, the emissive or glow simulation color, the object's specular highlights such as a bright spot reflecting the Sun on a car's hood, and any transparency. Colors in 3D are the same as those used on the Web—red, green, and blue. Though 3D colors span between 0 and 1, the Web often uses a hexadecimal number from 0 x 00 through 0 x FF. In the preceding code, the diffuse color used is 0.9 red, 0.6 green, and 0.3 blue for a light orange color.
The box also shows some shading with its brightest sides facing us. This is because there is a default headlight in the scene, which is positioned in the direction of the camera. This scene has no camera defined; in this case, a default camera will be inserted at the position (0, 0, 10), which is 10 units towards us along the z axis and points towards the origin (0, 0, 0). If you run this program (which you should), you will be able to rotate the viewpoint (camera) around the origin with the default headlight attached to the viewpoint. We will address lighting later, as lighting is a very important and complex part of 3D graphics.
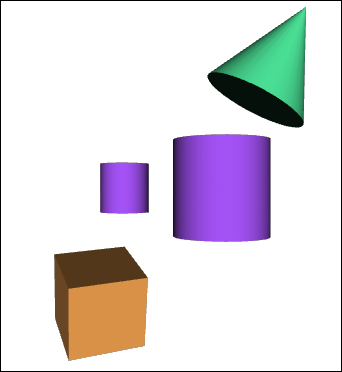