Let's look at some of the screen-shots and get a little bit more detail about each of the games from the book. We will go in to further detail and explanation as we start each project.
The first game we build will allow us to introduce some key Java beginner's topics. Code comments, variables, operators, methods, loops, generating random numbers, if
, else
, switch
and a brief introduction to object-oriented programming. We will also see how to communicate with the Android OS, detect screen touches, draw simple graphics, detect the screen resolution and handle different screen sizes. All this will be used to build a simpler variation of the classic Minesweeper type game. Here is one of the screens from that game.
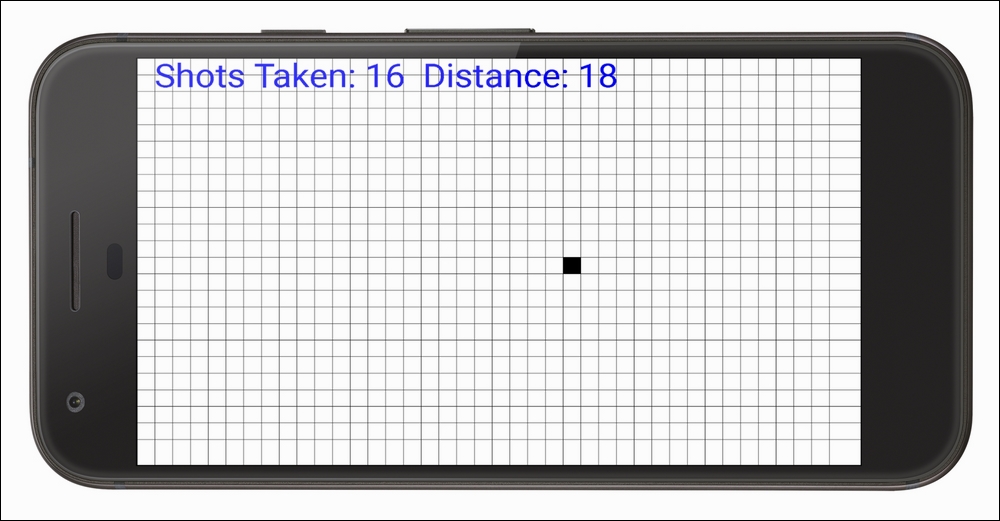
This will be a tap-to-shoot game where the player has to guess the position of the sub', then refine their next shot based on the "sonar" report of the previous shot.
For the second project, we will slightly increase the complexity and move on to 60 frames per second smoothly animated Pong clone. The Java topics covered include classes, interfaces, threads, try
-catch
blocks, method overloading vs. overriding and a much deeper look at object-oriented programming including writing and using our own classes. This project will also leave the reader competent with understanding the game loop, simple bouncing physics, playing sound effects and detecting game object collisions. Here is a picture of the simple but still a step-up Pong game.
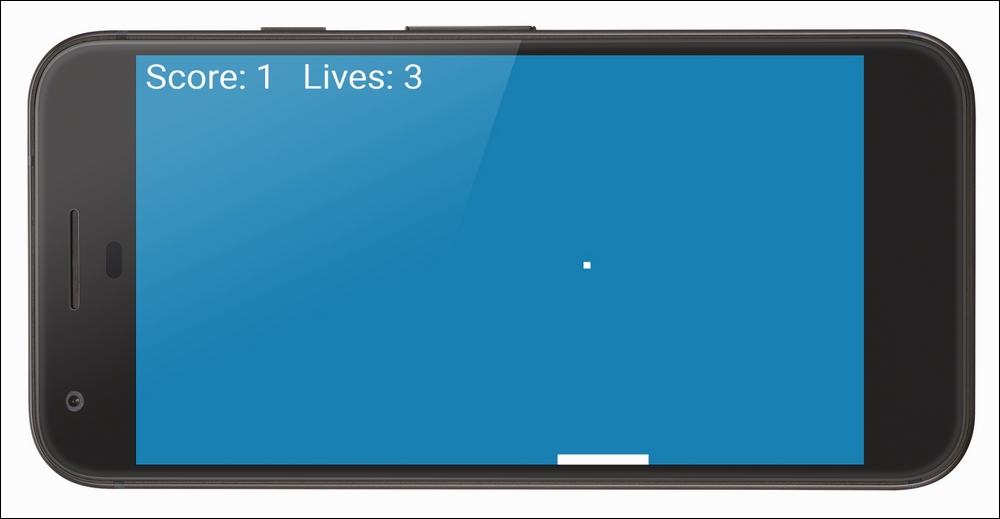
If the Pong game doesn't seem very busy, then the next game will not only be the exact opposite but will also giv e you the knowledge to improve the first two games.
In this project, we will meet Bob. In this Bullet Hell game, Bob will be a static image that can teleport at will anywhere on the screen to avoid the ever-growing number of bullets. In the final game we will also animate Bob, so he can run and jump around an explorable scrolling world. This short implementation will enable us to learn about Java arrays, Bitmap graphics and measuring time. We will also see how we can quite simply use Java arrays alongside what we have already learned about classes to spawn vast numbers of objects in our games.
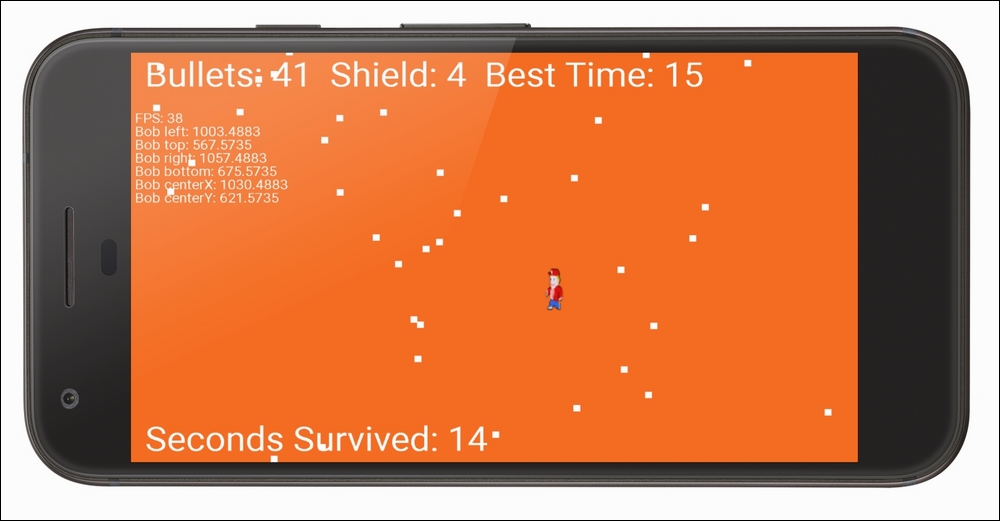
The objective of Bullet Hell is to survive for as long as possible. If a bullet is about to hit Bob the player can teleport him by tapping the desired destination on the screen, but each teleport spawns more bullets.
This game is a remake of the classic that has been enraging gamers for decades. Guide your snake around the screen and gaining points by eating apples. Each time you eat an apple your snake gets longer and harder to keep alive. In this project we learn about Java ArrayList
, enhanced for
loop, the Stack, the Heap and the Garbage collector (seriously- it's a thing) and we will also see how to make our games multilingual. Hola!
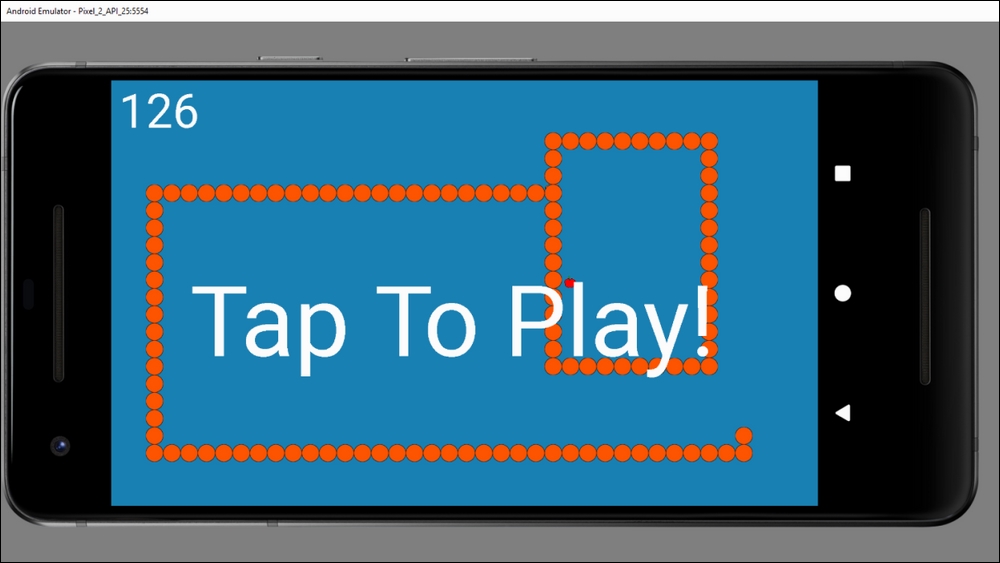
The game ends when the snake bumps into the edge of the screen or ends up eating part of his own body.
This project, technically speaking, is a big step up from Snake. We will learn how to handle multiple different alien types with unique behaviour, appearance and properties. Other features of the game include rapid fire lasers, scrolling background, persistent high score and a cool star-burst particle system explosion effect.
In this project we are introduced to Java and game programming patterns which are key to writing complex games with manageable code. We will learn about and use the Entity-Component pattern, Strategy pattern, Factory pattern and Observer pattern.
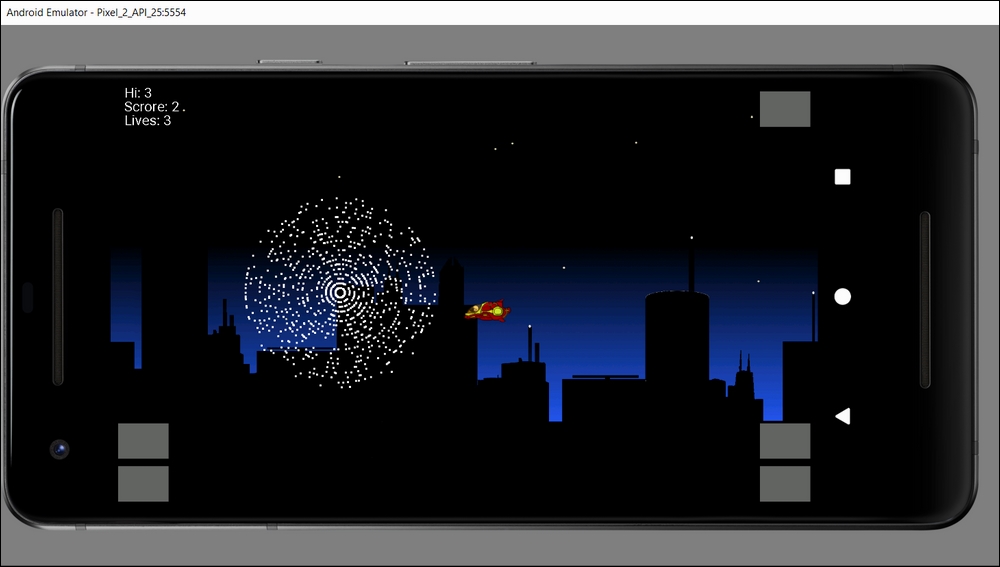
The techniques learned in this project are vital if you want to design your own games while structuring your Java code in a way that allows your games to become more complex and yet keep the code manageable.
In this project Bob makes a second appearance. The game will be a time trial where the player must get from the start point to the exit in the fastest time possible. There will be deadly jumps, moving platforms to navigate, fire pits and collectible coins. Every coin the player fails to collect will add a time penalty.
In this project we learn about the concept of a moveable camera that tracks the part of the game-world that needs to be shown to the player at any given moment. We will also learn some new Java patterns and reinforce the vital knowledge from the previous chapter. Furthermore, we will see how we can design levels as text layouts and then load them in code as playable levels.
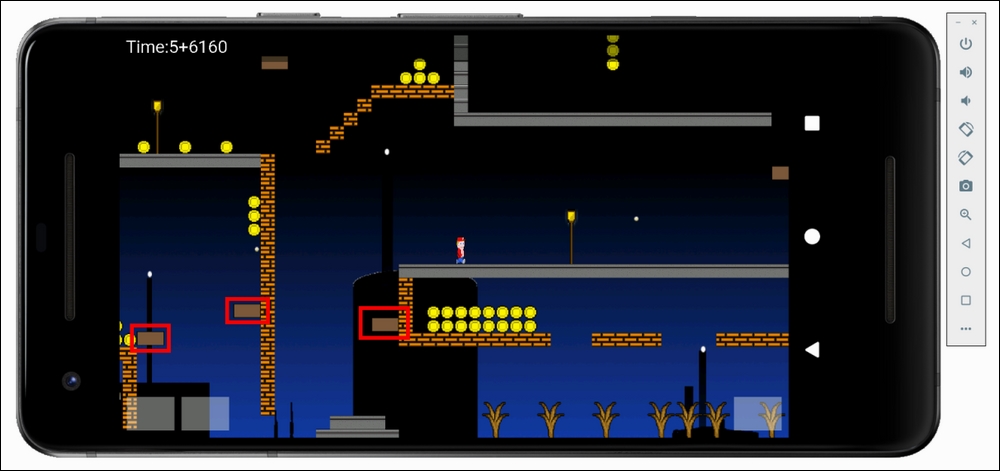
There are three levels and the player will choose the level they want to play from a home-screen which will also show the fastest times for each level.
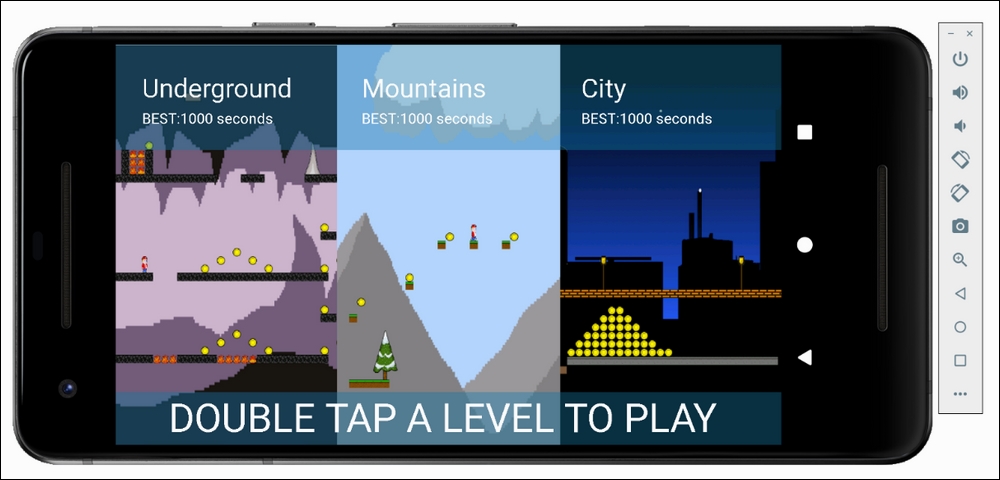
Let's learn a little about how Java and Android work.