Activities and other application components, such as services, are declared in the AndroidManifest XML file. Declaring an activity is how we tell Android about how the activity can be requested, and what code to run when it is requested. For example, an application will usually indicate that at least one activity should be visible as a desktop icon and serve as the main entry point to the application.
As with most recipes, we will be using the Eclipse IDE. If you have not done so already, start up Eclipse and ensure that you have installed the ADT Plugin.
Android projects are built against a target platform or API level. Here we have used API level 8, which corresponds to the Android 2.2 platform (FroYo). It is quite possible to use any level for this task but if you intend to make use of the 'holographic' UI you will need to look at the recipe about optimizing for 3.0 in Chapter 2, Layouts.
The Eclipse Android project wizard is as good a place to start building an application as any and it will automatically generate a manifest file that includes a basic activity declaration:
Run the project wizard. From the Eclipse File menu select New and then Android Project.
Enter the details of your project as you can see in the next screenshot and click on Finish:
Open up the manifest file from the Package Explorer, and then click on the AndroidManifest.xml tab at the bottom to display the code that the IDE has produced.
Within the
<activity>
element, find the following attributes:android:name=".DeclaringAnActivity" android:label="@string/app_name"
Edit the code so that it matches the following snippet:
<activity android:name=".DeclaringAnActivity" android:label="Welcome to the Android 3.0 Cookbook" android:screenOrientation="portrait"> ... </activity>
Run the application on a device or emulator. The title bar and screen orientation now reflect the changes that we have made. If you have not done this before, instructions can be found at http://developer.android.com/guide/developing/building/building-eclipse.html.
Note
Note that the use of string literals, as in "Welcome to the Android 3.0 Cookbook", is not considered good practice, as it makes translation next to impossible. String constants should be defined in a separate XML file; a literal is used here (and elsewhere in the book) only to simplify examples.
An activity represents a single task that the user can perform, such as editing some text or selecting a media file from a list. Each of our activities must be declared in the AndroidManifest
XML file, which resides in the root directory of the project.
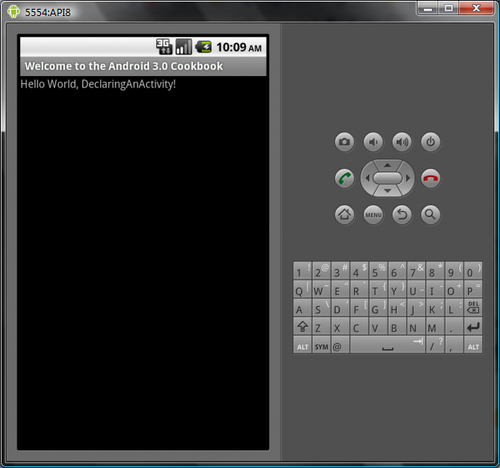
The project wizard provides us with two basic attributes:
The name DeclaringAnActivity refers to the Java subclass that will contain our activity's methods and fields.
The label app_name acts as a title for our application. It is displayed on the title bar of the device at runtime and also as the text under the application icon.
We also added an attribute of our own, screenOrientation
, which does exactly what you might expect it to.
The manifest is used to control an activity's start-up state and to apply features such as themes, or as just demonstrated, screen orientation. As we will see later though, most attributes can be set and changed dynamically through Java code as well.
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.PacktPub.com. If you purchased this book elsewhere, you can visit http://www.PacktPub.com/support and register to have the files e-mailed directly to you.