The Android application model can be seen as a service-oriented one, with activities as components and intents as the messages sent between them. Here, an intent is used to start an activity that displays the user's call log, but intents can be used to do many things and we will encounter them throughout this book.
To keep things simple, we are going to use an intent object to start one of Android's built-in activities, rather than create a new one. This only requires a very basic application, so start a new Android project with Eclipse and call it ActivityStarter
or something like that.
We are going to edit the Java subclass responsible for the main activity: the one declared in the manifest file. This class extends the activity class, and by overriding its onCreate()
method we can introduce code that will be executed when the application is first launched:
Using the Package Explorer, open the Java file inside the
src
folder of the project. It will have the same name as the activity, entered when the project was created:Add a new method to the class, similar to this one:
void startActivity() { Intent myIntent = new Intent(); myIntent.setAction(Intent.ACTION_CALL_BUTTON); startActivity(myIntent); }
Now, call this method from the
onCreate()
method so that it executes when the application is launched:@Override public void onCreate(Bundle state) { super.onCreate(state); setContentView(R.layout.main); startActivity(); }
Save and run the project. The application now displays the user's call log.
If this generates an error message, it may be that the correct libraries have not been imported. To use intents we have to import the relevant library, which can be done with
import android.content.Intent
; however it's easy to get Eclipse to import any missing libraries simply by pressing Shift + Ctrl + O.Press the back button on the device (or emulator) to see that the call log activity was actually called from our original main activity.
Intents operate as asynchronous messages sent between application components and they are used to activate services and broadcast receivers as well as activities. Intents are passive data structures that provide an infrastructure for our activities and other components.
The onCreate()
method is called as soon as the activity starts and so calling our startActivity()
method from within it means that we are immediately taken to the call log activity. More often than not we would use a button or menu item to perform such an action, and we haven't done so in order to simplify the demonstration and make it easier to incorporate in your own application.
Again, note that this project was built against Android 2.2 (API level 8) but this choice was arbitrary as the libraries used have been available since Android 1.5 and you should, ideally, build against the target device that you are testing on.
The previous example required only an action to be set but most intent objects make use of a setData()
method as well as the setAction()
method used.
Replace the setAction()
statement in the example with these two lines:
myIntent.setAction(Intent.ACTION_VIEW); myIntent.setData(android.provider.MediaStore.Images.Media.INTERNAL_CONTENT_URI);
This will open the device's image gallery when run and utilize both data and action parts of the intent.
Eclipse's auto-complete function allows us to explore Android's other baked-in activities. Simply start entering the code here and then scroll through the lists presented:
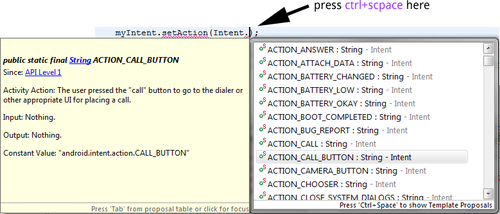
If the drop-down list fails to appear, press Ctrl + Space but note that when components share methods you may well see actions that correspond to other classes such as services or broadcasts, although the inline documentation is quite thorough and will mention when specific data or extra parameters are required.
To start an activity from a menu selection, see the recipe Handling menu selections in Chapter 4, Menus.