Unity provides an alternative way to display text in 3D via the TextMesh
component. While this is really suitable for a text-in-the-scene kind of situation (such as billboards, road signs, and generally wording on the side of 3D objects that might be seen close up), it is quick to create, and is another way of creating interesting menus or instructions scenes, and the like.
In this recipe, you'll learn how to create a scrolling 3D text, simulating the famous opening credits of the movie Star Wars, which looks something like this:
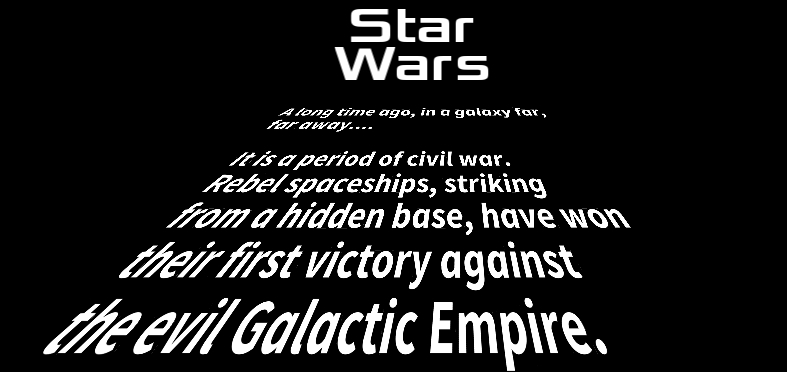
For this recipe, we have prepared the fonts that you need in a folder named Fonts
, and the text file that you need in a folder named Text
, in the 1362_01_04
folder.
To display perspective 3D text, follow these steps:
Create a new Unity 3D project (this ensures that we start off with a Perspective camera, suitable for the 3D effect that we want to create).
In the Hierarchy panel, select the Main Camera item, and, in the Inspector panel, set its properties as follows: Camera Clear Flags to solid color, Field of View to 150. Also set the Background color to black.
Import the provided
Fonts
folder.In the Hierarchy panel, add a UI | Text game object to the scene – choose menu: GameObject | UI | Text. Name this GameObject as
Text-star-wars
. Set its Text Content as Star Wars (with each word on a new line). Then, set its Font toXolonium Bold
, and its Font Size to50
. Use the anchor presets in Rect Transform to position this UI Text object at the top center of the screen.In the Hierarchy panel, add a 3D Text game object to the scene – choose menu: GameObject | 3D Object | 3D Text. Name this GameObject
Text-crawler
.In the Inspector panel, set the Transform properties for GameObject Text-crawler as follows: Position (
0
,-300
,-20
), Rotation (15
,0
,0
).In the Inspector panel, set the Text Mesh properties for GameObject Text-crawler as follows:
Paste the content of the provided text file,
star_wars.txt,
into Text.Set Offset Z =
20
, Line Spacing =0.8
, and Anchor = Middle centerSet Font Size =
200
, Font =SourceSansPro-BoldIt
When the scene is made to run, the Star Wars story text will now appear nicely squashed in 3D perspective on the screen.
You have simulated the opening screen of the movie Star Wars, with a flat UI Text object title at the top of the screen, and 3D Text Mesh with settings that appear to be disappearing into the horizon with 3D perspective 'squashing'.
There are some details that you don't want to miss.
With a few lines of code, we can make this text scroll in the horizon just as it does in the movie. Add the following C# script class, ScrollZ,
as a component to GameObject Text-crawler:
using UnityEngine; using System.Collections; public class ScrollZ : MonoBehaviour { public float scrollSpeed = 20; void Update () { Vector3 pos = transform.position; Vector3 localVectorUp = transform.TransformDirection(0,1,0); pos += localVectorUp * scrollSpeed * Time.deltaTime; transform.position = pos; } }
In each frame via the Update()
method, the position of the 3D text object is moved in the direction of this GameObject's local up-direction.
Learn more about 3D Text and Text Meshes in the Unity online manual at http://docs.unity3d.com/Manual/class-TextMesh.html.