This recipe illustrates how to create an interactive UI Slider, and execute a C# method each time the user changes the Slider value.
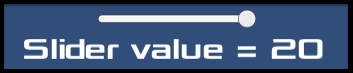
To create a UI Slider and display its value on the screen, follow these steps:
Create a new 2D project.
Add a UI Text GameObject to the scene with a Font size of
30
and placeholder text such asslider value here
(this text will be replaced with the slider value when the scene starts).In the Hierarchy panel, add a UI | Slider game object to the scene—choose the menu: GameObject | UI | Slider.
In the Inspector tab, modify settings for the Rect Transform to position the slider on the top-middle part of the screen and the text just below it.
In the Inspector tab, set the Min Value of the slider to
0
, the Max Value to20
, and check the Whole Numbers checkbox, as shown here:Create a C# script class called
SliderValueToText
, containing the following code, and add an instance as a scripted component to the GameObject called Text:using UnityEngine; using System.Collections; using UnityEngine.UI; public class SliderValueToText : MonoBehaviour { public Slider sliderUI; private Text textSliderValue; void Start (){ textSliderValue = GetComponent<Text>(); ShowSliderValue(); } public void ShowSliderValue () { string sliderMessage = "Slider value = " + sliderUI.value; textSliderValue.text = sliderMessage; } }
Ensure that the Text GameObject is selected in the Hierarchy. Then, in the Inspector view, drag the Slider GameObject into the public Slider UI variable slot for the
Slider Value To Text (Script)
scripted component, as shown here:Ensure that the Slider GameObject is selected in the Hierarchy. Then, in the Inspector view, drag the Text GameObject into the public None (Object) slot for the Slider (Script) scripted component, in the section for On Value Changed (Single).
Note
You have now told Unity to which object a message should be sent each time the slider is changed.
From the drop-down menu, select SliderValueToText and the
ShowSliderValue()
method, as shown in the following screenshot. This means that each time the slider is updated, theShowSliderValue()
method, in the scripted object, in GameObject Text will be executed.When you run the scene, you will now see a slider. Below it, you will see a text message in the
Slider value = <n>
form.Each time the slider is moved, the text value shown will be (almost) instantly updated. The values should range from
0
(the leftmost of the slider) to20
(the rightmost of the slider).Note
The update of the text value on the screen probably won't be instantaneous, as in happening in the same frame as the slider value is moved, since there is some computation involved in the slider deciding that an On Value Changed event message needs to be triggered, and then looking up any methods of objects that are registered as event handlers for such an event. Then, the statements in the object's method need to be executed in sequence. However, this should all happen within a few milliseconds, and should be sufficiently fast enough to offer the user a satisifyingly responsive UI for interface actions such as changing and moving this slider.
You have added to the Text GameObject a scripted instance of the SliderValueToText
class.
The Start()
method, which is executed when the scene first runs, sets the variable to be a reference to the Text component inside the Slider item. Next, the ShowSliderValue()
method is called, so that the display is correct when the scene begins (the initial slider value is displayed).
This contains the ShowSliderValue()
method, which gets the value of the slider. It updates the text displayed to be a message in the form: Slider value = <n>
.
You created a UI Slider GameObject, and set it to be whole numbers in the 0-20 range.
You added to the UI Slider GameObject's list of On Value Changed event listeners the ShowSliderValue()
method of the SliderValueToText
scripted component. So, each time the slider value changes, it sends a message to call the ShowSliderValue()
method, and so the new value is updated on the screen.