58. Introducing type pattern matching for instanceof
Can you name the shortcomings of the following classical snippet of code (this is a simple code used to save different kinds of artifacts on a USB device)?
public static String save(Object o) throws IOException {
if (o instanceof File) {
File file = (File) o;
return "Saving a file of size: "
+ String.format("%,d bytes", file.length());
}
if (o instanceof Path) {
Path path = (Path) o;
return "Saving a file of size: "
+ String.format("%,d bytes", Files.size(path));
}
if (o instanceof String) {
String str = (String) o;
return "Saving a string of size: "
+ String.format("%,d bytes", str.length());
}
return "I cannot save the given object";
}
You’re right…type checking and casting are burdensome to write and read. Moreover, those check-cast sequences are error-prone (it is easy to change the checked type or the casted type and forget to change the type of the other object). Basically, in each conditional statement, we do three steps, as follows:
- First, we do a type check (for instance,
o instanceof File
). - Second, we do a type conversion via cast (for instance,
(File) o
). - Third, we do a variable assignment (for instance,
File file =
).
But, starting with JDK 16 (JEP 394), we can use type pattern matching for instanceof to perform the previous three steps in one expression. The type pattern is the first category of patterns supported by Java. Let’s see the previous code rewritten via the type pattern:
public static String save(Object o) throws IOException {
if (o instanceof File file) {
return "Saving a file of size: "
+ String.format("%,d bytes", file.length());
}
if (o instanceof String str) {
return "Saving a string of size: "
+ String.format("%,d bytes", str.length());
}
if (o instanceof Path path) {
return "Saving a file of size: "
+ String.format("%,d bytes", Files.size(path));
}
return "I cannot save the given object";
}
In each if
-then
statement, we have a test/predicate to determine the type of Object o
, a cast of Object o
to File
, Path
, or String
, and a destructuring phase for extracting either the length or the size from Object o
.
The piece of code, (o instanceof File file
) is not just some syntactic sugar. It is not just a convenient shortcut of the old-fashioned code to reduce the ceremony of conditional state extraction. This is a type pattern in action!
Practically, we match the variable o
against File file
. More precisely, we match the type of o
against the type File
. We have that o
is the target operand (the argument of the predicate), instanceof File
is the predicate, and the variable file
is the pattern or binding variable that is automatically created only if instanceof File
returns true
. Moreover, instanceof File file
is the type pattern, or in short, File file
is the pattern itself. The following figure illustrates this statement:
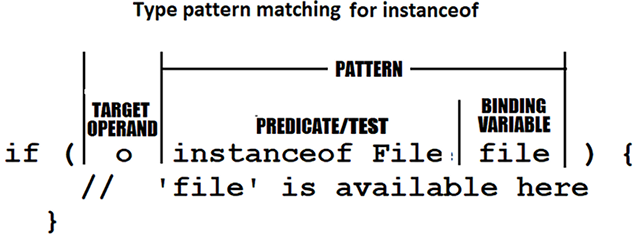
Figure 2.32: Type pattern matching for instanceof
In the type pattern for instanceof
, there is no need to perform explicit null
checks (exactly as in the case of plain instanceof
), and no upcasting is allowed. Both of the following examples generate a compilation error in JDK 16-20, but not in JDK 14/15/21 (this is weird indeed):
if ("foo" instanceof String str) {}
if ("foo" instanceof CharSequence sequence) {}
The compilation error points out that the expression type cannot be a subtype of pattern type (no upcasting is allowed). However, with plain instanceof
, this works in all JDKs:
if ("foo" instanceof String) {}
if ("foo" instanceof CharSequence) {}
Next, let’s talk about the scope of binding variables.