In the previous two recipes, we have seen how not having sufficient memory can be a problem to us. However, until now, we have had no control over how much memory is assigned and what is assigned to each memory address. Using pointers, we can address this issue. In my opinion, pointers are the single most important topic in C++. If your concept of C++ has to be clear, and if you are to become a good developer in C++, you must be good with pointers. Pointers can seem very daunting at first, but once you get the hang of it, pointers are pretty easy to use.
In this recipe, we will see how easy it is to work with pointers. Once you are comfortable using pointers, we can manipulate memory and store references in memory quite easily:
Open Visual Studio.
Create a new C++ project.
Select Win32 Console Application.
Add a source file called
main.cpp
or anything that you want to name the source file.Add the following lines of code:
#include <iostream> #include <conio.h> using namespace std; int main() { float fCurrentHealth = 10.0f; cout << "Address where the float value is stored: " << &fCurrentHealth << endl; cout << "Value at that address: " << *(&fCurrentHealth) << endl; float* pfLocalCurrentHealth = &fCurrentHealth; cout << "Value at Local pointer variable: "<<pfLocalCurrentHealth << endl; cout << "Address of the Local pointer variable: "<<&pfLocalCurrentHealth << endl; cout << "Value at the address of the Local pointer variable: "<<*pfLocalCurrentHealth << endl; _getch(); return 0; }
One of the most powerful tools of a C++ programmer is to manipulate computer memory directly. A pointer is a variable that holds a memory address. Each variable and object used in a C++ program is stored in a specific place in memory. Each memory location has a unique address. Memory addresses will vary depending on the operating system used. The amount of bytes taken up depends on the variable type: float = 4 bytes, short = 2 bytes:
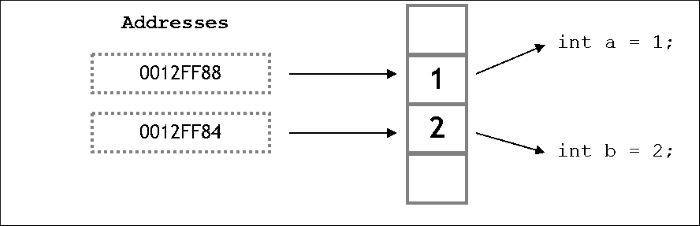
Pointers and memory storage
Each location in the memory is 1 byte. The pointer pfLocalCurrentHealth
holds the address of the memory location that has stored fCurrentHealth
. Hence, when we display the contents of the pointer, we get the same address as that of the address containing the fCurrentHealth
variable. We use the &
operator to get the address of the pfLocalCurrentHealth
variable. When we reference the pointer using the *
operator, we get the value stored at the address. Since the stored address is same as the address storing fCurrentHealth
, we get the value 10
.
Let us consider the following declarations:
const float* pfNumber1
float* const pfNumber2
const float* const pfNumber3
All of these declarations are valid. But what do they mean? The first declaration states that pfNumber1
is a pointer to a constant float. The second declaration states that pfNumber2
is a constant pointer to a float. The third declaration states that pfNumber3
is a constant pointer to a constant integer. The key differences between references and these three types of const pointers are listed here:
const
pointers can be NULLA reference does not have its own address, whereas a pointer does
The address of a reference is the actual object's address
A pointer has its own address and it holds as its value the address of the value it points to