Using the new await keyword and coroutines
The yield
keyword has been removed and replaced by the await
keyword. You can use await
with coroutines or signals. It pauses the function it is in and waits for a signal to be emitted, or if a called coroutine is finished, it then resumes the function where it was originally called.
Getting ready
For this recipe, create a new scene by clicking + to the right of the current Scene tab and adding Node2D. Select Save Scene As and name it Await
.
How to do it…
We will go through a very simple example of how await
works with a coroutine using a button as a character dialogue box. When the button is clicked, it skips to the next character dialogue box:
- In the new scene named
Await
that you have created, add a Button node and make it big enough to see the text that we are going to place in it with code. - Add a script named
Await
to Node2D and delete all of the default lines except line 1. - Let’s use
@onready
and create a variable calledbutton
to reference our Button node:1 extends Node2D 2 3 @onready var button = $Button
- On line 5, we will create a new function called
game_dialogue()
and call it in the_ready()
function:4 5 func _ready(): 6 game_dialogue() 7 8 func game_dialogue(): 9 button.text = "Dialogue text." 10 print("In the game_dialogue function.") 11 var next_dialogue = await skip_dialogue() 12 if next_dialogue: 13 print("At the end of game_dialogue function.")
- On line 15, we created a new function called
skip_dialogue()
:15 func skip_dialogue(): 16 print("Now in the skip_dialogue() function.") 17 await button.button_up 18 button.text = "New dialogue text." 19 print("At the end of skip_dialogue function." 20 return true
- Now click the Run the current scene button or hit the F6 key.
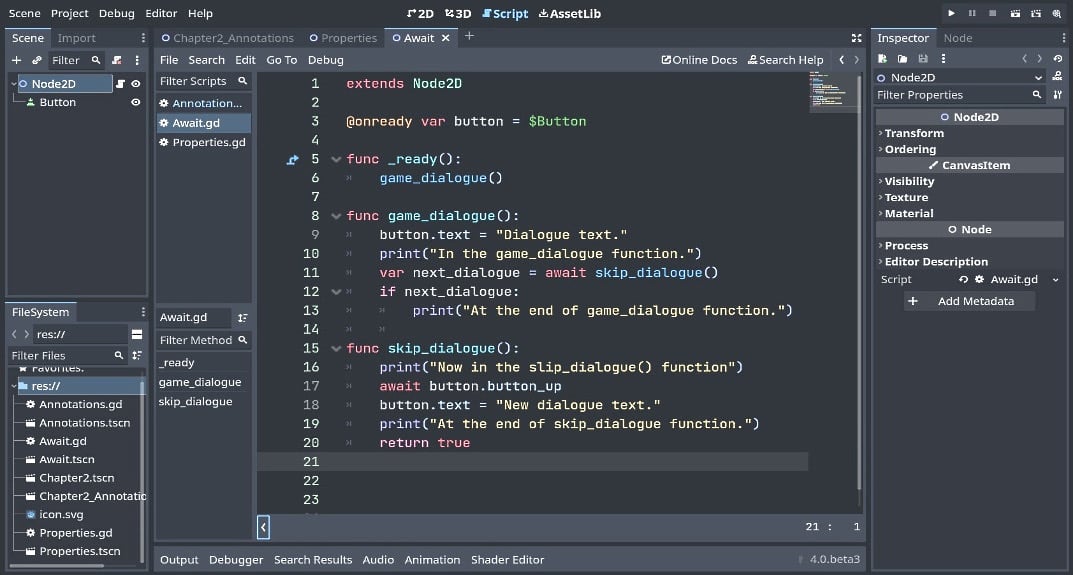
Figure 2.5 – GDScript for steps 3 to 5 (the code for Await.gd)
How it works…
We added a Button node and made it big enough for us to read the text on the button that we will code in later. Then we added a script called Await
to Node2D and deleted all of the default lines except line 1.
We used @onready
with the button
variable so we could load the reference when the _ready()
function was called.
We created a function called game_dialogue()
. In line 9, we added text to the button. In line 10, we printed that we were in this function so we could see it in the console to see how the await
keyword works. In line 11, we declared a variable called next_dialogue
equal to the skip_dialogue()
function written in step 5.
This is where the program will pause and go to the coroutine of the skip_dialogue()
function. In lines 12–13, we have an if
statement that checks that next_dialogue
is true
, and if so, then prints that we are back in the game_dialogue()
function. For it to be true
, the skip_dialogue()
function has to run and return true
.
We created the function called skip_dialogue()
. In line 16, we printed to the console that we were now in this function. In line 17, we waited for the user to click the button. In line 18, we changed the text on the button. In line 19, we printed to the console that we were at the end of the skip_dialogue()
function. In line 20, we return true
so that when we go back to the game_dialogue()
function, we can print the last print
statement.
We selected Run the current scene.
You see In the game_dialogue function.
and Now in the skip_dialogue() function.
on the console before you click the button. The default text on the button is Dialogue text.
. After you click the button, you see At the end of skip_dialogue function.
and At the end of game_dialogue function.
on the console and the text on the button is New
dialogue text.
.