No battle plan survives first contact with the enemy
There’s an old saying: if you fail to plan, you plan to fail. Only the rankest amateur would dive into a project IDE-first without at least considering how the project ought to be structured. The typical first steps might involve roughing out a package and object structure, or maybe designing the structure of a relational database that will persist the data used by our software. Someone who’s got a few projects under their belt might even draw some diagrams using the Unified Modeling Language (UML).
We begin by taking a set of user stories and we shape our code into something that on the surface meets the requirements in front of us. Soon, we’re in an agile groove swing. We’ve achieved velocity! We create a feature, show it to the customer, get feedback, revise, and continuously deliver. That’s usually how the troubles begin.
Our first major anti-pattern, the stovepipe system, comes from the seminal book on the subject, AntiPatterns, by Brown, et al., which I’ve listed as suggested reading at the end of this chapter.
The Stovepipe system
Once upon a time, in just about any industrialized society, people heated their homes and cooked using a cast-iron potbelly stove. These stoves burned coal or wood for fuel. Over time, the exhaust vent for the stove, called the stovepipe, since it was literally a pipe sticking out of the stove, would build up with corrosive deposits, which led to leaky stovepipes. The fumes from a burning stove are potentially life-threatening within a small, enclosed space.
Here’s what an actual stovepipe looks like:
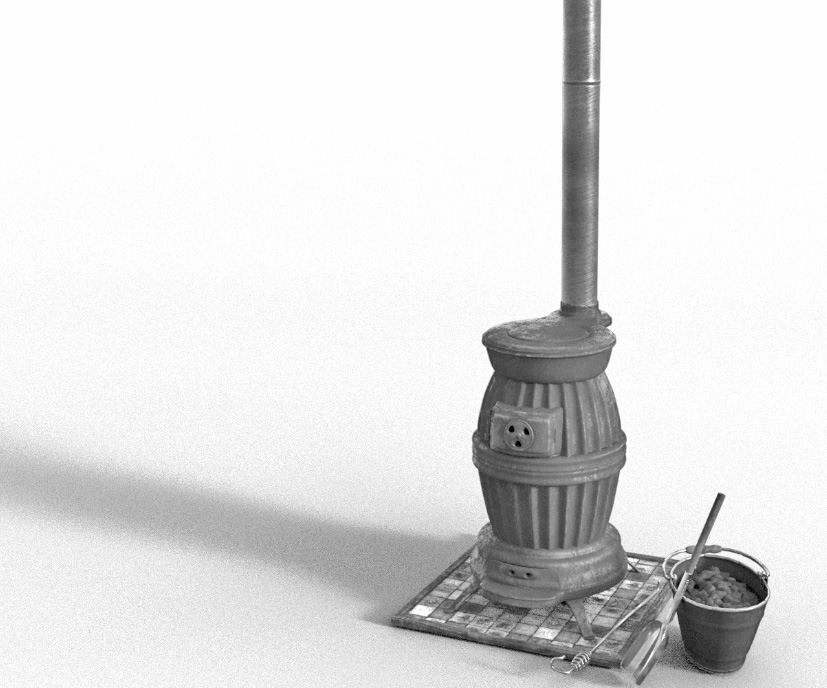
Figure 1.1 – A stove with a stovepipe.
The stovepipe required constant maintenance to prevent asphyxiation. This was usually done by the owner of the stove, who was unlikely to be a stove repair professional. The stove was repaired using tools and materials that were readily available. This made for very ad hoc patch jobs, rather than clean, well-thought-out repairs done with original equipment manufacturer (OEM) grade materials and the proper tools.
Now, think about how this might relate to a software project. The initial release is designed with great care, with an implementation that perfectly matches the design. The natural tendency during software maintenance is to fix things quickly and get the patched version released and out the door. As with our amateurish stove repairs, our analysis of the holes in the software design and implementation are perfunctory and incomplete. There is pressure to solve this quickly. Everybody is watching you. Every minute the application is down costs the company money and you risk losing the employee of the month parking spot.
This happens to everyone and everyone generally caves to human frailties. You then implement the quickest, easiest thing you can think of – it’s done and the patch is out the door. The crisis is over.
Or is it? Dun dun dun! Small ad hoc fixes have a negative cumulative effect over time, referred to as technical debt, just as the corrosive deposits on a stovepipe do. How can you tell whether the systems that you’re working on are stovepipe systems? Let’s explore the following:
- Stovepipe systems are monolithic by their very nature. It is not easy to get data in or out of this kind of system, and integrating software built this way into a larger enterprise architecture is cumbersome or impossible.
- Stovepipe systems are very brittle. When you make one of these small ad hoc repairs, you generally find that the fix breaks other parts of the application. Usually, this isn’t discovered until after the breaking fix has been released.
- Stovepipe systems can’t be easily extended as new business requirements emerge. When a project starts, you’re given a set of requirements. You build the software that meets those requirements. After it’s released, a new feature is requested that you couldn’t possibly have predicted. You realize that there’s no way to implement that feature without redesigning the whole app. Anytime you’re tempted to throw out the baby with the bathwater and just start over, you’re working on a stovepipe system.
- Stovepipe systems built on component architectures are generally incapable of sharing those components with other enterprise applications. The level of code reuse between projects is very low.
- Stovepipe systems are often found on projects with high turnover. This makes sense. You start a new job, replacing the last developer, and you feel pressure to get something working quickly to show your new boss that hiring you wasn’t a huge mistake. You do your best to piece something together to fix a problem. You have no knowledge of the existing architecture or what’s been tried, and perhaps failed, in the past. Now amplify this by considering two or three further re-staffing efforts, with several months between each new hire’s start dates.
- Stovepipe systems are often indicated when the development team is using new or unfamiliar technologies, stacks, or languages. Given that the same pressure to produce something quickly exists, while the team is simultaneously required to work with tools and languages that they’ve never used before, this leads to the same pattern of just getting something working and released. You will also encounter stovepipe systems in start-ups, corporate acquisitions, and mergers for these same reasons.
Does any of this sound familiar? Naturally, we’re not talking about anything you’ve ever written! Isn’t this just a little bit reminiscent of code you’ve seen other people write? Maybe your competitors? Maybe your students? Even if you own up to writing a stovepipe system, don’t beat yourself up. It is far and away the most popular pattern in software development today. Sometimes, a stovepipe system is fine. Remember, not every physical edifice needs to be supported by fluted ivory columns, and there’s a very legitimate argument to be made for getting the software to market and worrying about the rest later. However, if your objective is to build software that’s still useful and profitable 10 years or more down the road, keep reading. We’ll have those stovepipes replaced with functional, modular, well-constructed systems in no time.