The Golden Hammer
Another important antipattern you should learn to recognize is generally a product of some marketing organization or salesperson in a company outside your own. It happens when some killer app, framework, infrastructure component, or tool is presented as the panacea for all your software development woes. It slices, it dices, it makes julienne fries, and it automatically refactors itself while speeding up the execution of your code.
The antipattern is described as the Golden Hammer. Behold a fully-rendered CGI representation in Figure 1.2:
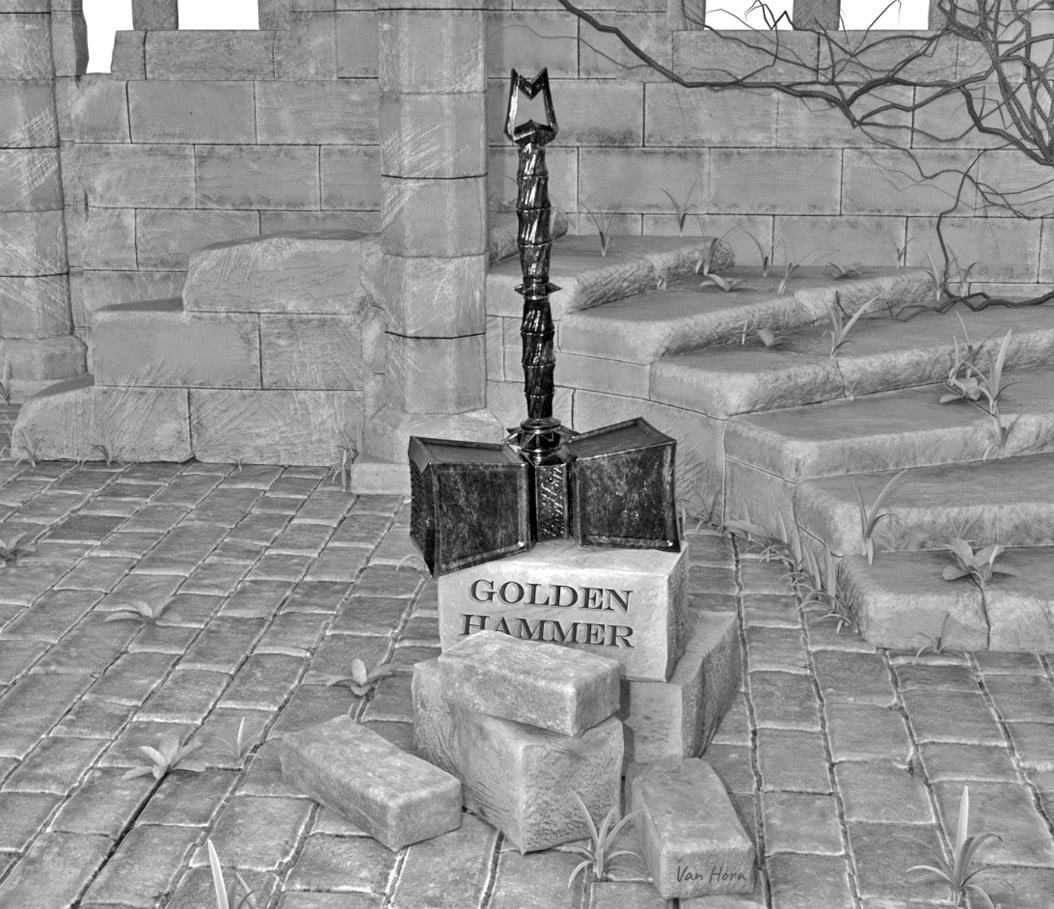
Figure 1.2 – When you’re given a golden hammer, everything is a nail.
Silicon snake oil salespeople will visit you, take you out to someplace fancy, and try to convince you that the database tool, platform, or whatever-as-a-service (WaaS) that they’re selling can be the entire basis for your company’s software. Consider Microsoft SQL Server for a minute. At its most basic, SQL Server is a relational database. It stores your data in tables that you can query. Related tables of data can be joined and filtered allowing a developer who understands the Structured Query Language (SQL) to produce reporting data in any format or configuration. This is a common functionality found in every relational database tool from Microsoft Access and SQLite to Oracle and Microsoft SQL Server. Since SQL is a standardized language, offering this basic functionality is little more than table stakes. Just so we understand each other, all puns are intended.
So, how could Microsoft expect to charge money for something that you can get for free in open source offerings such as MySQL and PostgreSQL? Granted, SQL Server got started before they did when there were fewer rivals in the marketplace, but SQL Server is one of the most popular platforms for managing data today. This is because SQL Server’s value contribution doesn’t end with tabular data storage. As the product has grown over the years, new features and ancillary tools have been added. You have the ability to load and analyze data in novel and sophisticated ways using SQL Server Analysis Services. SQL Server Reporting Services allows you to create reports using SQL and then present those reports graphically to whoever might need them by emailing the reports as PDFs. It also allows users to access the report on the server and play around with the data without needing to know SQL or have access to the underlying code.
There are supported workflows for working with AI and machine learning projects using R and Python, and you can make bits of code in C# that process in the database such as a native stored procedure. SQL Server Integration Services allows you to ingest and publish data to a variety of different databases, software services, and industry formats. This leads to the ability to integrate your software and services with your business partners and customers.
In short, if you tried hard enough, you could probably write a great deal of an application, if not an entire one, solely using SQL Server’s ecosystem. SQL Server is the Golden Hammer. Every problem now looks as if it’s something that can be solved with SQL Server. I want to point out that I am not vilifying SQL Server. It’s a reliable and cost-effective set of tools. I go out of my way to recommend it at parties and my advice is always well received. Note to self: find better parties. I picked on SQL Server because I’ve seen it happen with this particular tool. If you spend too much time reading the marketing material for SQL Server, it would be easy for you to walk away with the same conclusion: that SQL Server is all you need. Maybe it is, but you should only make that decision after you understand the Golden Hammer antipattern, lest you wind up painting yourself into a technological corner.
The Golden Hammer also emerges when a developer learns about some technology that was unknown to them before. They use it. They like it. They’re rewarded for it in the form of fast or novel solutions to a problem. Since that worked out so well, and since they’ve gone to the effort of adding a new skill to their skill set, they try to use that tool or technique to solve every problem that they encounter.
Once, I took over a project that was in trouble. The lead programmer on a small team was being let go and most of his team left shortly after I replaced him. Interpersonal drama aside, I set out to understand the new project and the business domain by going through the existing code base.
I asked around and, as it turned out, the original staff on the project were with a consulting firm. The firm sent a couple of their ace developers over to meet and gather requirements. Upon seeing the prototypes that the client had themselves produced in Excel, using Visual Basic for Applications (VBA), the consultants concluded that they could produce real code in a real language and have a fully converted program running in under a month.
Two years went by with no usable deliverables. The ace developers either grossly underestimated the prototype they were working with or overestimated their capabilities. I think it was a little of both. Most developers look down their noses at VBA. I’ll admit that I used to, even though I’ve written quite a bit of VBA code. The consultants erroneously concluded that VBA is simplistic. They believed anything written in VBA would involve a trivial amount of effort to convert to a language as powerful as C#, backed by the equally powerful .NET Framework and SQL Server.
After a few months with very little progress, the consulting firm pulled their ace developers off the project to work on something else and the project was staffed entirely by junior developers.
Given the antipatterns we’ve covered so far, you can already see where this story is headed. I inherited this code after two and half years without a viable release. As I went through the existing code, I was able to see where the junior developers had encountered some tool or technique. It was as if I was looking at rings on a tree:
.jpg)
Figure 1.3 – The effects of new developers discovering a golden hammer are analogous to tree rings in their code.
You can tell exactly where they have learned that a stored procedure can be used in SQL Server because, from that point forward, the business logic suddenly moved out of the code and into the database. This is usually a bad idea. It’s often done because you can change the business rules without compiling and publishing a new executable, allowing you to make minor or major adjustments. This is roughly akin to working on the engine of an airplane while it’s flying at 1,261 knots (about 1,453 mph or 2,336 km/h) at 30,000 feet (9,144 m).
Somewhere else, you can tell they have read a book on patterns because the code changes. Suddenly, everything has interfaces and uses the factory pattern, which we’ll cover later in Chapter 3. Some of this was good. I could see that they were improving. However, a lot of it was someone picking up a new hammer and using it to bang everything around it into the shape of something useful. This was evident mainly because they were never given a chance to go back and refactor their earlier work. They used different techniques at different points in time. They weren’t always using the best tool for the job, but they were motivated by the forces we discussed earlier. They did their best with what they had, as with our stovepipe repair jobs.